URI dialling in UC infrastructure is a good thing to have for a number of reasons. For example, if your dial plan is not flexible enough and the number of digits used for DNs is insufficient to cover all endpoints. In that case moving toward URI will give you more flexibility (in case you don’t want to enable access codes).
Another reason that sounds tempting is the expansion of Cisco Jabber usage: some of my users don’t want a hardware phone any more and ask to give them the opportunity to make and receive call with Jabber only. In that case I can leave them with Directory URI only which is equal to their email, which is nice.
Moreover, URI dialling is essential when making B2B calls.
So here I’m going to cover some potential cases of URI dialling with CUCM and VCS/Expressway
1) Cisco video codecs
SX10 and SX20 codecs are very powerful devices. If they are not provisioned by CUCM or VCS they can dial via URI out-of-the-box provided SIP and H.323 protocols are on and there is no SIP proxy of H.323 gatekeeper configured.
For example, if you dial reception@example.com from a SX20 it will do the necessary DNS lookups for example.com domain and send SIP INVITE to the corresponding IP.
But in most cases codecs are managed by CUCM and all SIP INVITES are send to it. In that case you need to set up intracluster/intercluster URI dialling on CUCM and B2B dialling through collaboration edge VCS/Expressway.
I still keep H.323 protocol enabled (Configuration->System configuration->Networkservices->H323 ON) for SX20 to be capable of calling H323 destinations on its own.
2) Cisco Jabber
Jabber calling capabilities are managed and provisioned by CUCM by using special type of devices (i.e, CSF for Cisco Jabber for windows). If you want to make calls to URI from Jabber you should enable this function in jabber-config.xml
- download jabber-config.xml from CUCM TFTP by navigating to http://%5BIP address of CUCM node with TFTP running]:6970/jabber-config.xml
- in the policies section change EnableSIPURIDialling parameter to true
- upload updated jabber-config.xml to TFTP via Cisco Unified Operating System Administration -> Software updates -> TFTP File Management -> Upload file (use / directory)
- restart TFTP service
After restarting Cisco Jabber your devices will be able to use URIs.
3) Intracluster URI dialling
There is a document on cisco.com describing the process of enabling intracluster URIs. I want to expand it a little bit.
If you have you user account synchronized with LDAP, their URI could be imported to CUCM as a part of this synchronization. Navigate to Cisco Unified CM Administration ->End User -> select a user.
In End User Configuration window there should be a populated Directory URI filed. Then scroll down and find Directory Number Associations.
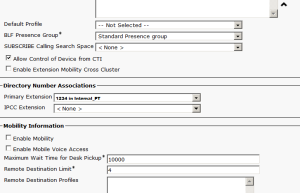
The drop-down Primary Extension will be populated if an end user has a control of a device with DN configured (see Device Information -> Controlled Devices in this window). So when you choose a DN user’s Direcotry URI get’s assosiated with this DN and is automatically assigned to a partition defined by System -> Enterprise Parameters -> End User Parameters -> Directory URI Alias Partition. Be sure to add this partition to a correspondent CSS.
Another option is to define custom (up to 5 different) URIs under DN configuration. Navigate to a device -> Association (DN association section on the left), scroll down to Directory URIs section and add one (and choose a partition). If you have already completed first option via End User Configuration window, in that case Directory URI will show up here with a green OK sign.

And don’t forget to check Use Fully Qualified Domain Name in SIP Requests checkbox in all SIP profiles used by Cisco Jabber devices and codecs.
Now you can use URI for calling between Jabber and video codecs inside you CUCM cluster
4) Intercluster URI dialling
This task is accomplished with ILS,which I think deserves a separate post.
In part 2 I’m going to cover B2B URI dialling both inbound and outbound.